Preparing our Project
No lecture description Lesson locked purchase
Description
Learn to create your very own C compiler from scratch. In this course we develop a compiler that compiles a subset of the C Programming Language. By the time you finish all modules of this course you will be able to compile C programs that use pointers, structures, unions, arrays, functions, for loops, while loops. do while loops, if statements, switches and much more! This course includes all course modules!
Our compiler also has a preprocessor macro system allowing you to include header files and create definitions just like you would in any C file.
Your compiler is advanced enough to use the GCC standard library so we are able to call C functions from our compiler. Your compiler will be able to compile the C programming language.
This course does not rely on any frameworks we do everything from scratch to ensure the best possible learning experience for students
Module 1
In module 1 of this course we load our C source file that we wish to compile, into memory. We create a lexer to preform lexical analysis on the source input which will convert the source code into a bunch of tokens that our compiler can easily understand. We then pass the tokens through a parser to produce an abstract syntax tree. An AST describes the C program in a logical way that makes it easier for our compiler to understand. For example for the expression 50 + 20 you will end up with a root expression node that has a left operand that has a node of value 50 and a right operand that has a node of value 20. Breaking down problems in this way makes it much easier to create compilers.
Module 2
In module 2 of this course we create a code generator that produces 32 bit Intel assembly language that can then be passed through an assembler to produce a program binary that we can run. We also in this module create a resolver system which is responsible for taking a complicated expression such as "a->b.c.e[50] = 50" and breaking it down into simple steps and rules that our code generator can then easily follow. This abstraction is essential to ensure that the code generator does not become over complex. With the use of a resolver system we can ensure the code base remains clean.
Module 3
In module 3 of this course we create a preprocessor and macro system. This preprocessor system allows us to include header files in our C programs and also use a variety of macro keywords such as "#define" "#ifdef" , "sizeof" and many more.
Module 4
In module 4 we build a semantic validator which validates our C code. A semantic validator ensures that we are not setting variables that do not exist or accessing structures that arent there.
This is the only video course in the world that shows you how to create a C compiler, come and learn today!
Requirements
-
You must have a basic experience of assembly language.
Who This Course is For
People with an interest in compiler design
People who are interested in assembly language
People who are interested in the C Programming language
What You Will be Learn
How to build a C compiler from scratch
Full understanding of stackframes and how assembly language is generared for a C source file
Complete Understanding of lexical analysis and parsing
Stronger Assembly language skills will be gained
Compiler Design
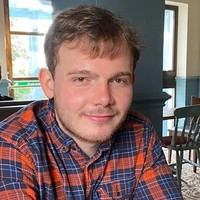
Dragon Zap Instructor
Daniel McCarthy is a seasoned software engineer, boasting an impressive career spanning over 14 years in the industry. Holding a Master's Degree in Advanced Computer Science from Cardiff Metropolitan University, his broad spectrum of experience encompasses everything from web development to complex compiler and interpreter development. Daniel has honed his skills in bootloader and kernel development. In testament to his proficiency in the field, he has designed two proprietary programming languages: Craft, a general-purpose language, and Marble, a web-focused language akin to PHP. Moreover, he has successfully developed compilers for the C programming language. A testament to his versatility, Daniel demonstrates proficiency in an extensive list of programming languages that includes C, C++, Java, x86 Assembly language, PIC assembly, SQL, PHP, HTML5, JavaScript, CSS, and of course, his own creations, Craft and Marble. His professional portfolio also includes the development of Linux kernel modules, a task he has executed with proficiency in a professional context. Currently, Daniel is channeling his wealth of experience and expertise into the education sector, with the aim of nurturing the next generation of professional software engineers.
Ask a question
Questions (5)
Amwtke Amwtke
1 year ago
Hi Daniel, may I ask when the third module will come out?
Daniel McCarthy
1 year ago
Hi Amwtka, it is currently being uploaded by our upload team, you will see it shortly.
Lane Beals
11 months ago
Does every part of your vector struct get used? I'm going to make my own as more C practice/preparation before continuing on with the course, and was wondering if I needed to implement every part. There are common important parts of a vector type, such as the beginning, end, push back, insert, pop, etc that will need to be implemented. So I'm just wondering if there are any parts that are not necessarily required.
Daniel McCarthy
11 months ago
Hello, Not everything gets used but you dont need to write anything anyway, its a quick copy and paste if you watch from the start of the course youll find it, I show you where to find the vector files. We dont implement that functionality ourselves as its not related to compiler dev Thanks
Daniel McCarthy
11 months ago
If you want to make your own vector to train your C your welcome but I recommend only using the vector code as seen on this course for the actual compiler course otherwise if something goes wrong your going to not know if it was your vector or not
Tabish Raza
9 months ago
Hey, Dan I have watched this video like 3 times yet not able to understand a single thing from this video... I don't know why but it feels like you should re-take this video and take a little time to explain what you are actually doing... As you said earlier I learned makefile, learned about header in c, and function pointer... Yet things are totally going over me... Please can you take time to explain things going on in this video?
Daniel McCarthy
9 months ago
Hi Tabish, I understand your frustration as building a compiler is not easy however stick with it and all will be fine. I wont be retaking the video as its correct. Please let me know what you had problems with so I can further assist you. What exactly did you struggle with Thanks Dan
Tabish Raza
9 months ago
Yes, your video is absolutely correct I agree... I was just suggesting that it would be better if you could explain a little bit more what you are doing in your videos... Anyways, First thing I would like to understand is Makefile... I have read and understand basic of it but the what OBJECT variable is doing doesn't make sense to me and even INCLUDE variable Apart from that I understand that "all" and "clean" is target but what does "./build/compiler.o: ./compiler.c gcc ./compiler.c ${INCLUDES} -o ./build/compiler.o -g -c" this do? Thanks Tabish
Daniel McCarthy
8 months ago
I do understand the reason it is not covered is because your expected to come to the course understanding those things as compiler development is a very advanced topic so your expected to be proficient before starting the course which is why I suggested for you to research more on C and makefiles. The command you pointed out to me states a rule called ./build/compiler.o this rule states that compiler.c is the source file and should compile into ./build/compiler.o. By specifying it in the OBJECT variable and requireing it in the "all" label it will ensure compiler.o is compiled and it requires compiler.o to exist before the all label can continue. That is what the makefile is it basically defines rules on how a project should be compiled. This learning is essential and cant be rushed please go back and take your time and soon enough you will meet the requiements and be able to continue the course. Here is additional information for learning makefiles: https://makefiletutorial.com/ I have a course on C programming as well on this website which you can purchase if you choose. Additionally I have a course on assembly language as well all can be found at my teacher profile: https://dragonzap.com/teacher/daniel-mccarthy
Daniel McCarthy
8 months ago
These things cannot be covered in this particular course as this course is a compiler development course. Makefiles are more suited to a course tailored to learning C. I do actually teach them in my C programming course as well found at my teaching profile
Larry E. Dickey
4 months ago
Hi Dan. I've just about completed your kernel course on Udemy. What I'm wondering here, on this preparation lecture is; Can I use GCC the way we configured it for the kernel course, in this course ? Or, will that present a problem ? That's how I have GCC currently set up.
Daniel McCarthy
4 months ago
Hello Larry, Congratulations on finishing the kernel course. You will need to use the normal GCC compiler and not your cross build version. Run the command on Ubuntu "sudo apt install gcc" and then run the "gcc -v" command to see the current version. Ensure you use the "gcc" command and not your cross compiler build. Thanks Dan
Larry E. Dickey
4 months ago
Hi Dan. Thanks for the prompt response and the info regarding my question. I greatly appreciate it ! I had a feeling that the `cross build' version wouldn't be appropriate for building this compiler. I just wanted to be sure to verify that with you before I start in on this. Thanks again, Larry
Daniel McCarthy
4 months ago
Your very welcome Larry please reach out for any more questions have a great christmas and new year
Panayotis Mavromatis
3 months ago
Hi Dan, thank you for such an organized and principled approach to project layout. I feel safe following your lead on this. I have studied C, but have never attempted a large-scale project in that language. I feel a bit nervous not having unit tests around. Is this something you have found helpful in your work with C? If so, is there a unit test library for C that you would recommend? I am eager to add my own unit tests as I follow along. Many thanks in advance!
Daniel McCarthy
3 months ago
Hello, your very welcome. We do not do unit tests in the compiler project because the compiler its self is so large so building unit tests would be a waste of lesson time, the course as it stands is over 40 hours long so with unit tests that would easily add another 4-5 hours, for this reason the choice not to include unit tests was made. If you insist on making unit tests I have heard CUnit is useful. Many thanks Dan
Panayotis Mavromatis
3 months ago
Hi Dan, thank you for your quick reply. This is helpful. Yes, it makes sense re: lesson time. I may give CUnit a try.
Zachary Rowitsch
3 weeks ago
One that I have used for a bunch of projects is "Unity" by ThrowTheSwitch on github